Spring Mobility
Spring mobility is nothing but making a node to move in a path similar to that of an expanded circular coil spring. From the top or bottom point of view, the movements of the nodes will just look like circle mobility.
But if we view the scenario from any other direction, then it will show the node ascending or descending in a path similar to that of a circular coil spring, For example, in this example scenario, even though all the three planes seem to be roaming in a circular path, one plane (white) is descending to the ground while it is circulating in the sky.
About this Work
But, we can not simulate circular mobility as well as spring mobility using any of the existing models of ns-3. But sometimes, a simulation may need a node to move in a perfectly circular or an expanded, circular coil-spring like path. In our previous article, “Implementation of Circle Mobility Model for ns-3 and Visualizing it in 3D” we presented the idea of simulating circular mobility model under ns-3.
In this article, we present the idea of creating “SpringMobilityModel” by cloning and extending our previous “CircleMobilityModel” code.
In this tutorial, I show a new mobility model class implementation for simulating “spring mobility” under ns-3 using most of the ideas that we used in our previous circular mobility. You can see a typical coil spring to understand the mobility model that I am mentioning here.
Caution: This simulation only addresses the primitive problems in simulating and visualizing a 3D UAV/FANET Simulation and Mobility models. There are other aspects such as setting different routing protocols and different traffic types in such 3D UAV/FANET scenario. They are not addressed in this article. Further, there are some additional aspects needed to do a trace analysis on the simulated 3D UAV/FANET scenario; that are also not discussed here. Further, for simulating realistic movements and orientation of 3d nodes, we have to do some modifications in the core Netsimulyzer. That is also not described here.
The Main Idea of the Design of a New Mobility Model.
As I mentioned earlier, we can easily implement a new, simple mobility model by closing one of the existing mobility models and including the desired functionality in it. For our design of “SpringMobilityModel” I simply cloned our previous “CircleMobilityModel” and include the functionality needed to simulate spring mobility in it.
Even though a 3D visualization even 2D visualization is not at all needed while doing scholarly research work, while learning the fundamentals of a simulation, for better understanding, all of us just want to visualize the network that we are simulating. For that, we need a better visualization of the simulated network. In addition to that, the pictorial and video outputs of the simulated network will be useful to make one understand our ideas during our project or research presentations.
The “SpringMobilityModel” Class of our “spring-mobility-model.h” file
The following are the important member functions and variables that are declared in CircleMobilityModel class of the header files circle-mobility-model.h.
void SetParameters(const Vector &Origin, const double Radius, const double StartAngle, const double Speed, zChange);
virtual Vector DoGetPosition (void) const;
virtual void DoSetPosition (const Vector &position);
virtual Vector DoGetVelocity (void) const;
Vector m_position; //!< the constant position
Vector m_Origin;
double m_Radius;
double m_StartAngle;
double m_Speed;
Time m_lastUpdate
double m_zChange;
The Important functions of our “spring-mobility-model.cc” file
The following function SetParameters will be called from the simulation script to set the required parameters of the circular mobility.
The parameters to create circle mobility are:
- Origin of the Circle (in Meters)
- The radius of the Circle (in Meters)
- Starting Angle (in Degree),
- Speed (m/sec);
- and the Change in Z direction (m/sec);
void
SpringMobilityModel::SetParameters(const Vector &Origin, const double Radius, const double StartAngle, const double Speed, const double zchange)
{
m_Origin=Origin;
m_Radius=Radius;
m_StartAngle=StartAngle;
m_Speed=Speed;
m_zChange=zchange;
DoSetPosition(Origin);
NotifyCourseChange ();
}
The Function DoGetPosition
The following function will be called in regular intervals by the parent class of the mobility model and make the nodes move to position accordingly in an ns-3 simulation.
The following function will call NotifyCourseChange while setting the position of the node.
void
SpringMobilityModel::DoSetPosition (const Vector &position)
{
m_position = position;
m_lastUpdate = Simulator::Now ();
NotifyCourseChange ();
}
The following function will be called in regular intervals by the parent class of the mobility model so that we can get the velocity of the nodes in an ns-3 simulation.
Vector
SpringMobilityModel::DoGetVelocity (void) const
{
Time now = Simulator::Now ();
NS_ASSERT (m_lastUpdate <= now);
//m_lastUpdate = now;
double angle = m_StartAngle+ ((m_Speed/m_Radius) * now.GetSeconds());
double cosAngle = cos(angle);
double sinAngle = sin(angle);
return Vector ( -sinAngle * m_Speed, cosAngle * m_Speed,0);
}
The Components of the Simple 3D FANET ns-3 Simulation Script
The following code segment presents the important components of the ns-3 simulation script which is used to simulate and test our new spring mobility model. In this simulation, two aeroplane nodes will use circle mobility and another node will use the proposed spring mobility model.
Include the header files Needed
The following lines include the necessary header files. The Netsimulyzer ns-3 module should be installed in the ns-3 directory tree.
#include “ns3/core-module.h”
#include “ns3/mobility-module.h”
#include “ns3/netanim-module.h”
#include “ns3/netsimulyzer-module.h”
The following lines show the creation of nodes for this FANET network simulation.
NodeContainer CirclesUAV;
CirclesUAV.Create (2);
NodeContainer SpringUAV;
SpringUAV.Create (1);
Simulate Circle Mobility and Spring Mobility in nodes
The following section of code will do the important part of adding mobility model in the nodes.
MobilityHelper CircleMobility;
CircleMobility.SetMobilityModel (“ns3::CircleMobilityModel”);
CircleMobility.Install (CirclesUAV);
CirclesUAV.Get (0)->GetObject
CirclesUAV.Get (1)->GetObject
MobilityHelper SpringMobility;
SpringMobility.SetMobilityModel (“ns3::SpringMobilityModel”);
SpringMobility.Install (SpringUAV);
SpringUAV.Get (0)->GetObject
Specify the output File and Set Different 3D models for the Simulated FANET nodes
Add a Backbround Decoration
auto decoration1 = CreateObject
decoration1->SetAttribute (“Model”, StringValue(“HouseAndRoad.obj”));
decoration1->SetPosition ({0,0,0});
decoration1->SetAttribute (“Scale” , DoubleValue (2));
// decoration->SetAttribute (“Height”, OptionalValue
The 3D View of the simulated FANET in NetSimulyzer.
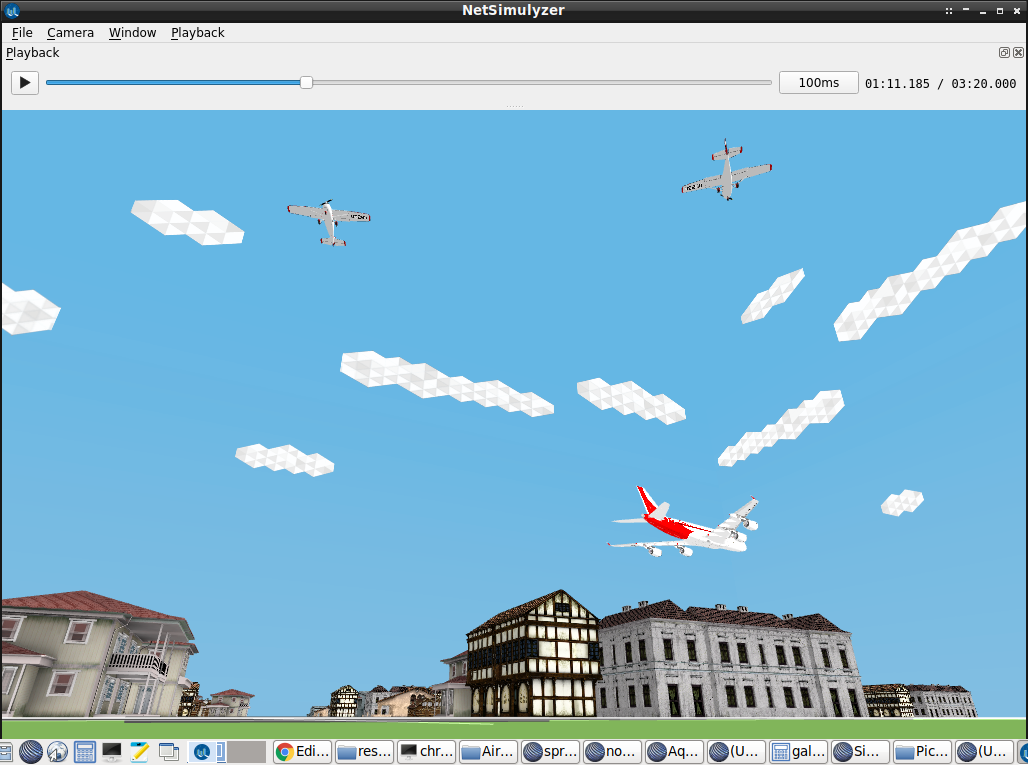
Configure standard NetAnim file and end the simulation
For comparison purposes, we create a NetAnim simulation file, which will only be capable of showing 2D of this 3D FANET scenario.
AnimationInterface anim (“SimpleNS3FANETCircleSpringMobility3D.xml”);
Simulator::Stop (Seconds (200));
Simulator::Run ();
Simulator::Destroy ();
The 2D visualization with NetAnim
The following NetAnim video output shows the Circle Mobility and Spring Mobility of the nodes. Of course we can not really understand the 3D motion of the Spring Mobility Model from this 2D video output.
The 3D Circle Mobility Model Demo
The following video shows the 3D output of the FANET simulation with CircleMobilityModel and SpringMobilityModel on NetSimulyzer. Under this 3D visualization, we can understand the difference in this two mobility models.
Note: The visualization of Packet Transmission and wave Propagation on Medium are not yet implemented in NetSimulyzer. Further, the movement tracking using lines is also not yet implemented in NetSimulizer. So we can only see the movement of nodes. But we can add “log events” to understand the events of transmission and reception through some text messages that will be displayed in a separate text window of NetSimulyzer.